Android 开发即时聊天工具 YQ :(三) 实现登陆功能
|
admin
2013年2月25日 14:19
本文热度 3740
|
前面socket基本通信完了,登陆界面也已经完成,下面就是重点了,实现登陆功能
服务器和客户端的代码当然不肯能用那个控制台的那个了,所以全部得重写,不过原理都一样,代码也差不多,都有注释,一看就明白。
先是登陆的Activity:
- public class LoginActivity extends Activity {
- protected void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.activity_login);
- Button btnLogin=(Button) findViewById(R.id.btn_login);
- btnLogin.setOnClickListener(new OnClickListener(){
- public void onClick(View arg0) {
- int account=Integer.parseInt(((EditText) findViewById(R.id.et_account)).getText().toString());
- String password=((EditText) findViewById(R.id.et_password)).getText().toString();
- login(account, password);
- }
- });
- }
-
- void login(int a, String p){
- User user=new User();
- user.setAccount(a);
- user.setPassword(p);
- user.setOperation("login");
- boolean b=new YQConServer().sendLoginInfo(user);
-
- if(b){
- try {
-
- "WHITE-SPACE: pre">
- } catch (IOException e) {
- e.printStackTrace();
- }
-
- Intent i=new Intent(this, MainActivity.class);
- startActivity(i);
- }else {
- Toast.makeText(this, "登陆失败!不告诉你为什么,", Toast.LENGTH_SHORT).show();
- }
- }
- }
public class LoginActivity extends Activity {
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
Button btnLogin=(Button) findViewById(R.id.btn_login);
btnLogin.setOnClickListener(new OnClickListener(){
public void onClick(View arg0) {
int account=Integer.parseInt(((EditText) findViewById(R.id.et_account)).getText().toString());
String password=((EditText) findViewById(R.id.et_password)).getText().toString();
login(account, password);
}
});
}
void login(int a, String p){
User user=new User();
user.setAccount(a);
user.setPassword(p);
user.setOperation("login");
boolean b=new YQConServer().sendLoginInfo(user);
//登陆成功
if(b){
try {
//发送一个要求返回在线好友的请求的Message
//---
} catch (IOException e) {
e.printStackTrace();
}
//转到主界面,
Intent i=new Intent(this, MainActivity.class);
startActivity(i);
}else {
Toast.makeText(this, "登陆失败!不告诉你为什么,", Toast.LENGTH_SHORT).show();
}
}
}
将登陆的信息封装到user中,user的operation用来判断该user包的类型,交由YQClient来发送到服务器。
客户端:
- public class YQClient{
- public Socket s;
-
- public boolean sendLoginInfo(Object obj){
- boolean b=false;
- try {
- s=new Socket();
- try{
- s.connect(new InetSocketAddress("10.0.2.2",5469),2000);
- }catch(SocketTimeoutException e){
-
- return false;
- }
- ObjectOutputStream oos=new ObjectOutputStream(s.getOutputStream());
- oos.writeObject(obj);
- ObjectInputStream ois=new ObjectInputStream(s.getInputStream());
- YQMessage ms=(YQMessage)ois.readObject();
- if(ms.getType().equals(YQMessageType.SUCCESS)){
-
-
- b=true;
- }else if(ms.getType().equals(YQMessageType.FAIL)){
- b=false;
- }
- } catch (IOException e) {
- e.printStackTrace();
- } catch (ClassNotFoundException e) {
- e.printStackTrace();
- }
- return b;
- }
- }
public class YQClient{
public Socket s;
public boolean sendLoginInfo(Object obj){
boolean b=false;
try {
s=new Socket();
try{
s.connect(new InetSocketAddress("10.0.2.2",5469),2000);
}catch(SocketTimeoutException e){
//连接服务器超时
return false;
}
ObjectOutputStream oos=new ObjectOutputStream(s.getOutputStream());
oos.writeObject(obj);
ObjectInputStream ois=new ObjectInputStream(s.getInputStream());
YQMessage ms=(YQMessage)ois.readObject();
if(ms.getType().equals(YQMessageType.SUCCESS)){
//创建一个该账号和服务器保持连接的线程
//---
b=true;
}else if(ms.getType().equals(YQMessageType.FAIL)){
b=false;
}
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return b;
}
}
在登陆成功后,将会新开一个线程和服务器保持连接,该线程将用来通信,
要捕获SocketTimeoutException异常,否则连接 不到服务器,app会无响应,这里设置2s服务器无响应,则连接服务器超时。
最后在看服务器端:
- public class YQServer {
- public YQServer(){
- ServerSocket ss = null;
- try {
- ss=new ServerSocket(5469);
- System.out.println("服务器已启动,正在监听5469端口...");
- while(true){
- Socket s=ss.accept();
-
- ObjectInputStream ois=new ObjectInputStream(s.getInputStream());
- User u=(User) ois.readObject();
- YQMessage m=new YQMessage();
- ObjectOutputStream oos=new ObjectOutputStream(s.getOutputStream());
-
- if(u.getOperation().equals("login")){
- int account=u.getAccount();
- boolean b=new UserDao().login(account, u.getPassword());
- if(b){
- System.out.println("["+account+"] 上线了!");
- m.setType(YQMessageType.SUCCESS);
- oos.writeObject(m);
-
-
- }else{
- m.setType(YQMessageType.FAIL);
- oos.writeObject(m);
- }
- }else if(u.getOperation().equals("register")){
-
- }
- }
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
-
- }
public class YQServer {
public YQServer(){
ServerSocket ss = null;
try {
ss=new ServerSocket(5469);
System.out.println("服务器已启动,正在监听5469端口...");
while(true){
Socket s=ss.accept();
//接受客户端发来的信息
ObjectInputStream ois=new ObjectInputStream(s.getInputStream());
User u=(User) ois.readObject();
YQMessage m=new YQMessage();
ObjectOutputStream oos=new ObjectOutputStream(s.getOutputStream());
if(u.getOperation().equals("login")){ //登录
int account=u.getAccount();
boolean b=new UserDao().login(account, u.getPassword());//连接数据库验证用户
if(b){
System.out.println("["+account+"] 上线了!");
m.setType(YQMessageType.SUCCESS);//返回一个成功登陆的信息包
oos.writeObject(m);
//单开一个线程,让该线程与该客户端保持连接
//---
}else{
m.setType(YQMessageType.FAIL);
oos.writeObject(m);
}
}else if(u.getOperation().equals("register")){
//注册
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
最后测试一下:
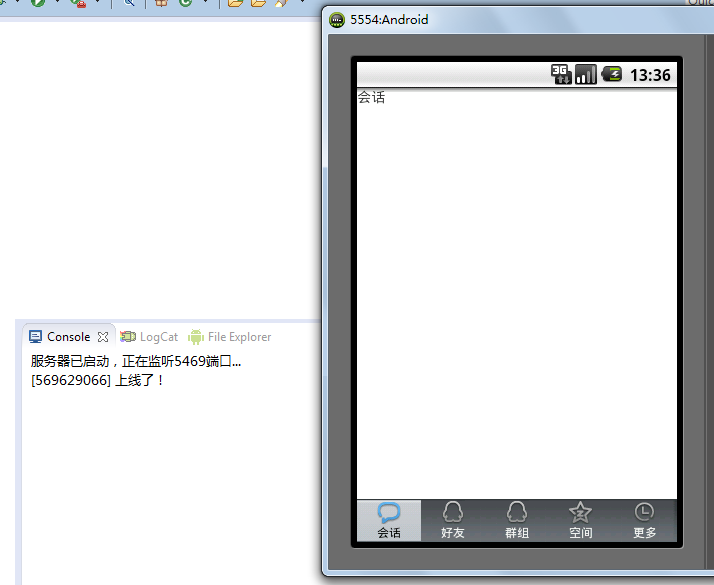
该文章在 2013/2/25 14:19:38 编辑过