C#WinForm利用Aspose.Cells实现DataGridView导出Excel
|
admin
2025年2月20日 19:34
本文热度 195
|
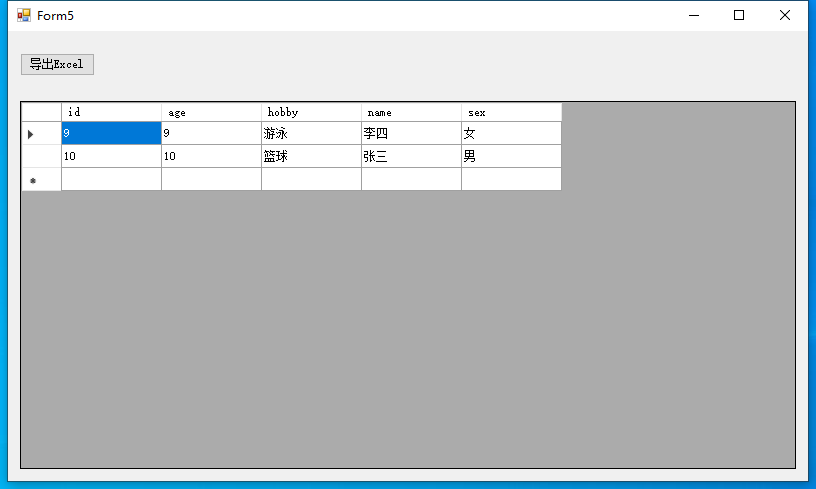
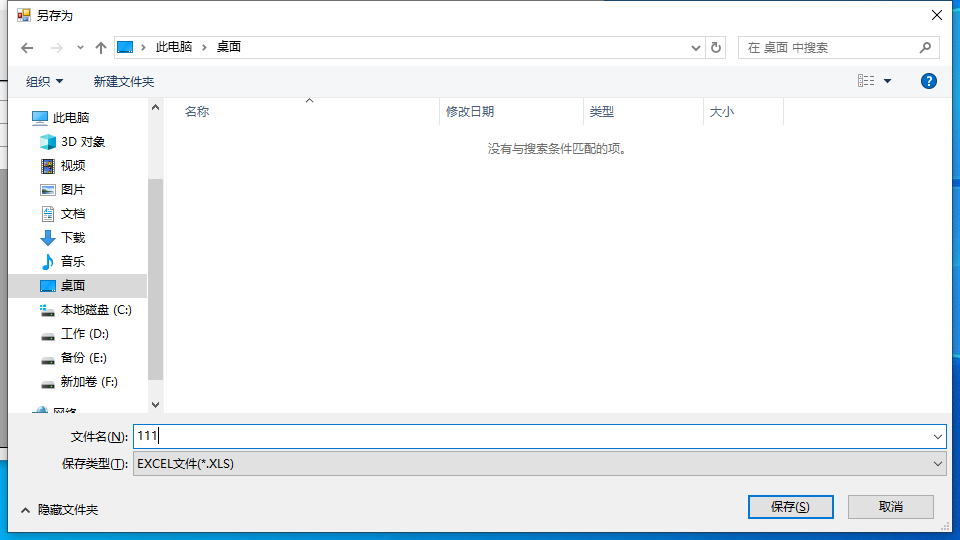
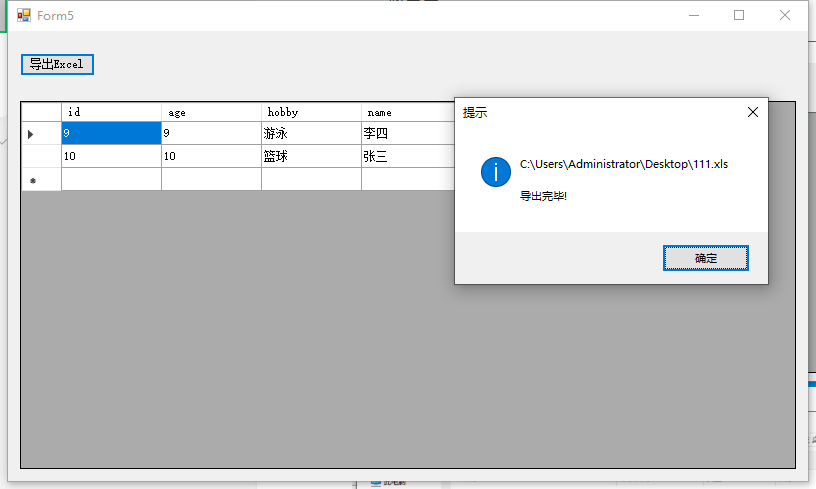
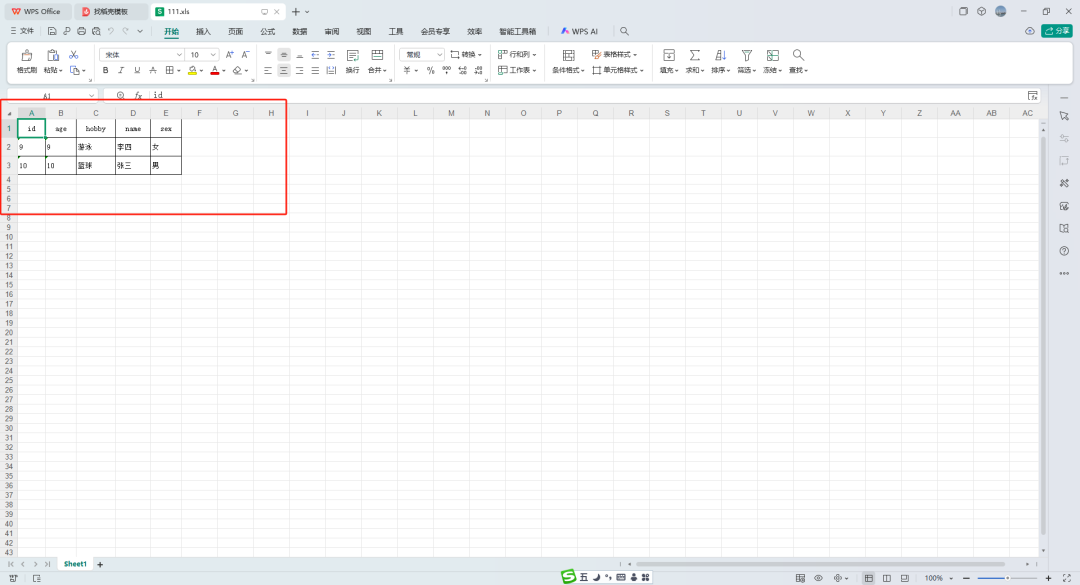
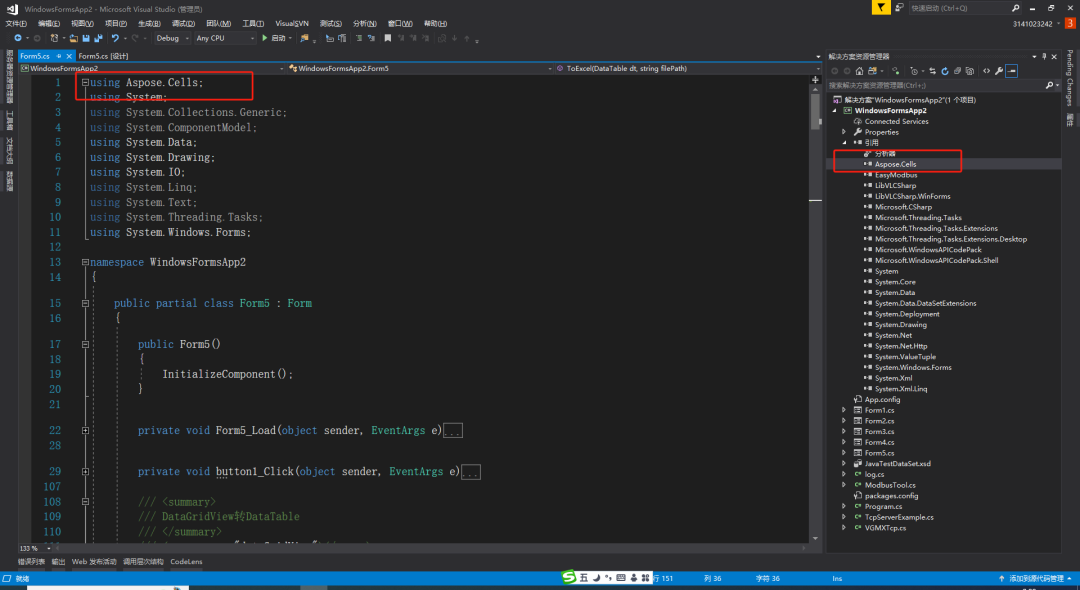
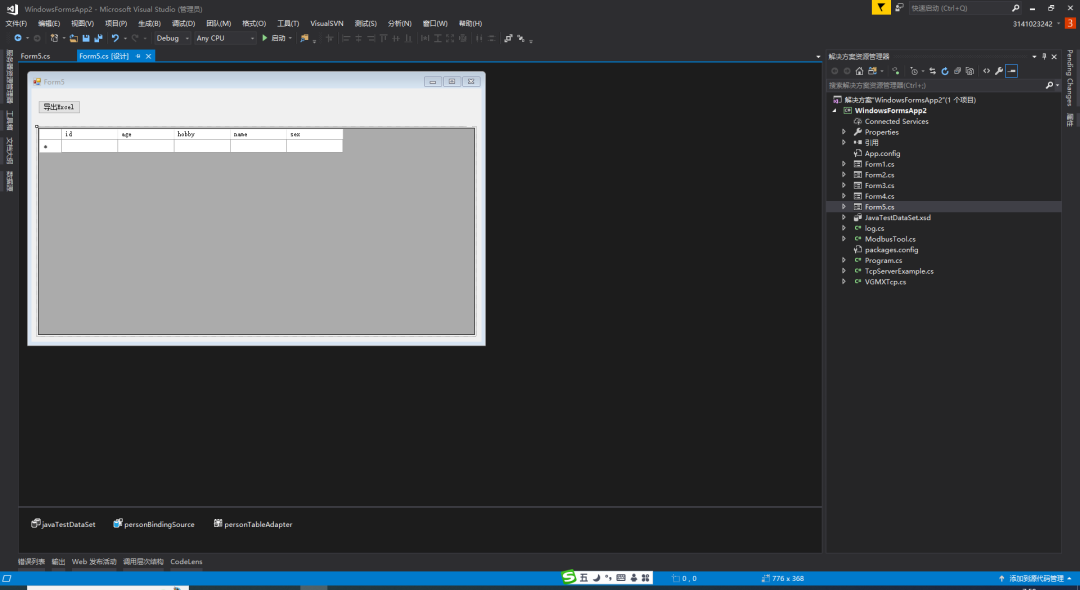
private void button1_Click(object sender, EventArgs e)
{
#region 验证可操作性
//申明保存对话框
SaveFileDialog dlg = new SaveFileDialog();
//默然文件后缀
dlg.DefaultExt = "xls ";
//文件后缀列表
dlg.Filter = "EXCEL文件(*.XLS)|*.xls ";
//默然路径是系统当前路径
dlg.InitialDirectory = Directory.GetCurrentDirectory();
string fileNameString = dlg.InitialDirectory + "\\printExcel.xls";
//打开保存对话框
if (dlg.ShowDialog() == DialogResult.Cancel) return;
//返回文件路径
fileNameString = dlg.FileName;
//验证strFileName是否为空或值无效
if (fileNameString.Trim() == " ")
{ return; }
//定义表格内数据的行数和列数
int rowscount = dataGridView1.Rows.Count;
int colscount = dataGridView1.Columns.Count - 1;
//行数必须大于0
if (rowscount <= 0)
{
MessageBox.Show("没有数据可供保存 ", "提示 ", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
//列数必须大于0
if (colscount <= 0)
{
MessageBox.Show("没有数据可供保存 ", "提示 ", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
//行数不可以大于65536
if (rowscount > 65536)
{
MessageBox.Show("数据记录数太多(最多不能超过65536条),不能保存 ", "提示 ", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
//列数不可以大于255
if (colscount > 255)
{
MessageBox.Show("数据记录行数太多,不能保存 ", "提示 ", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
//验证以fileNameString命名的文件是否存在,如果存在删除它
FileInfo file = new FileInfo(fileNameString);
if (file.Exists)
{
try
{
file.Delete();
}
catch (Exception error)
{
MessageBox.Show(error.Message, "删除失败 ", MessageBoxButtons.OK, MessageBoxIcon.Warning);
return;
}
}
#endregion
#region 导出Excel
var dataTableTemp = DataGridViewToDataTable(dataGridView1);
ToExcel(dataTableTemp, fileNameString);
#endregion
MessageBox.Show(fileNameString + "\n\n导出完毕! ", "提示 ", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
/// <summary>
/// DataGridView转DataTable
/// </summary>
/// <param name="dataGridView"></param>
/// <returns></returns>
public static DataTable DataGridViewToDataTable(DataGridView dataGridView)
{
DataTable dt = new DataTable();
foreach (DataGridViewColumn column in dataGridView.Columns)
{
dt.Columns.Add(column.HeaderText, typeof(string)); // 假设类型以string的方式添加
}
foreach (DataGridViewRow row in dataGridView.Rows)
{
if (!row.IsNewRow) // 忽略添加空行(通常最后一行是空行)
{
DataRow dataRow = dt.NewRow();
for (int i = 0; i < dataGridView.Columns.Count; i++)
{
dataRow[i] = row.Cells[i].Value;
}
dt.Rows.Add(dataRow);
}
}
return dt;
}
#region 数据表保存至Excel
/// <summary>
/// DataTable数据表保存至Excel
/// </summary>
/// <param name="dt">数据源</param>
/// <param name="filePath">文件完整路径</param>
public static void ToExcel(DataTable dt, string filePath)
{
string subTitle = string.Empty;
//新建工作簿
Workbook wb = new Workbook();
//新建工作表
Worksheet ws = wb.Worksheets[0];
ws.Name = dt.TableName;
//dt.Columns.Remove("Id");
int rowIndex = 0;
int colIndex = 0;
int colCount = dt.Columns.Count;
int rowCount = dt.Rows.Count;
ws.Cells.SetRowHeight(rowIndex, 25);//设置行高
//创建样式
Style style = wb.Styles[wb.Styles.Add()];//新增样式
style.HorizontalAlignment = TextAlignmentType.Center; //单元格内容的水平对齐方式文字居中
style.Font.Name = "宋体"; //字体
//style.Font.IsBold = true; //设置粗体
//style.Font.Color = Color.White;//设置字体颜色
style.Font.Size = 10; //设置字体大小
//style.ForegroundColor = Color.FromArgb(0, 196, 180); //背景色
style.Pattern = BackgroundType.Solid;
style.Borders[BorderType.TopBorder].LineStyle = CellBorderType.Thin;
style.Borders[BorderType.TopBorder].Color = Color.Black;
style.Borders[BorderType.BottomBorder].LineStyle = CellBorderType.Thin;
style.Borders[BorderType.BottomBorder].Color = Color.Black;
style.Borders[BorderType.LeftBorder].LineStyle = CellBorderType.Thin;
style.Borders[BorderType.LeftBorder].Color = Color.Black;
style.Borders[BorderType.RightBorder].LineStyle = CellBorderType.Thin;
style.Borders[BorderType.RightBorder].Color = Color.Black;
//列名的处理
for (int i = 0; i < colCount; i++)
{
ws.Cells[rowIndex, colIndex].PutValue(dt.Columns[i].ColumnName);
ws.Cells[rowIndex, colIndex].SetStyle(style);//给单元格关联样式
colIndex++;
}
Style style2 = wb.Styles[wb.Styles.Add()];//新增样式
style2.Font.Name = "宋体";//文字字体
style2.Font.Size = 10;//文字大小
style2.ShrinkToFit = true;
style2.VerticalAlignment = TextAlignmentType.Center;
style2.Borders[BorderType.TopBorder].LineStyle = CellBorderType.Thin;
style2.Borders[BorderType.TopBorder].Color = Color.Black;
style2.Borders[BorderType.BottomBorder].LineStyle = CellBorderType.Thin;
style2.Borders[BorderType.BottomBorder].Color = Color.Black;
style2.Borders[BorderType.LeftBorder].LineStyle = CellBorderType.Thin;
style2.Borders[BorderType.LeftBorder].Color = Color.Black;
style2.Borders[BorderType.RightBorder].LineStyle = CellBorderType.Thin;
style2.Borders[BorderType.RightBorder].Color = Color.Black;
rowIndex++;
for (int i = 0; i < rowCount; i++)
{
ws.Cells.SetRowHeight(rowIndex, 25);//设置行高
colIndex = 0;
for (int j = 0; j < colCount; j++)
{
if (dt.Columns[j].ColumnName == "净重(T)" || dt.Columns[j].ColumnName == "扣杂(T)" || dt.Columns[j].ColumnName == "标准水分%" || dt.Columns[j].ColumnName == "实测水分%" || dt.Columns[j].ColumnName == "扣水吨位(T)" || dt.Columns[j].ColumnName == "标准灰分%" || dt.Columns[j].ColumnName == "实测灰分%" || dt.Columns[j].ColumnName == "扣灰吨位(T)" || dt.Columns[j].ColumnName == "扣杂后净重(T)" || dt.Columns[j].ColumnName == "结算重量(T)" || dt.Columns[j].ColumnName == "结算单价(元/T)" || dt.Columns[j].ColumnName == "结算金额(元)")
{
ws.Cells[rowIndex, colIndex].PutValue((dt.Rows[i][j].ToString() == "" ? 0 : Math.Round(decimal.Parse(dt.Rows[i][j].ToString()), 2)).ToString("0.00"));
}
else
{
ws.Cells[rowIndex, colIndex].PutValue(dt.Rows[i][j].ToString() == "" ? null : dt.Rows[i][j].ToString());
}
if (i == rowCount - 1)
{
//style2.ForegroundColor = Color.Gray;
style2.Pattern = BackgroundType.Solid;
ws.Cells[rowIndex, colIndex].SetStyle(style2);//给单元格关联样式
}
else
{
style2.ForegroundColor = Color.White;
style2.Pattern = BackgroundType.Solid;
ws.Cells[rowIndex, colIndex].SetStyle(style2);//给单元格关联样式
}
colIndex++;
}
rowIndex++;
}
//设置所有列为自适应列宽
ws.AutoFitColumns();
for (int col = 0; col < colCount; col++)
{
ws.Cells.SetColumnWidthPixel(col, ws.Cells.GetColumnWidthPixel(col) + 20);
}
if (System.IO.File.Exists(filePath))
System.IO.File.Delete(filePath);
System.IO.FileStream fs = System.IO.File.Create(filePath);
fs.Close();
fs.Dispose();
wb.Save(filePath);
}
#endregion
阅读原文:原文链接
该文章在 2025/2/21 12:26:34 编辑过